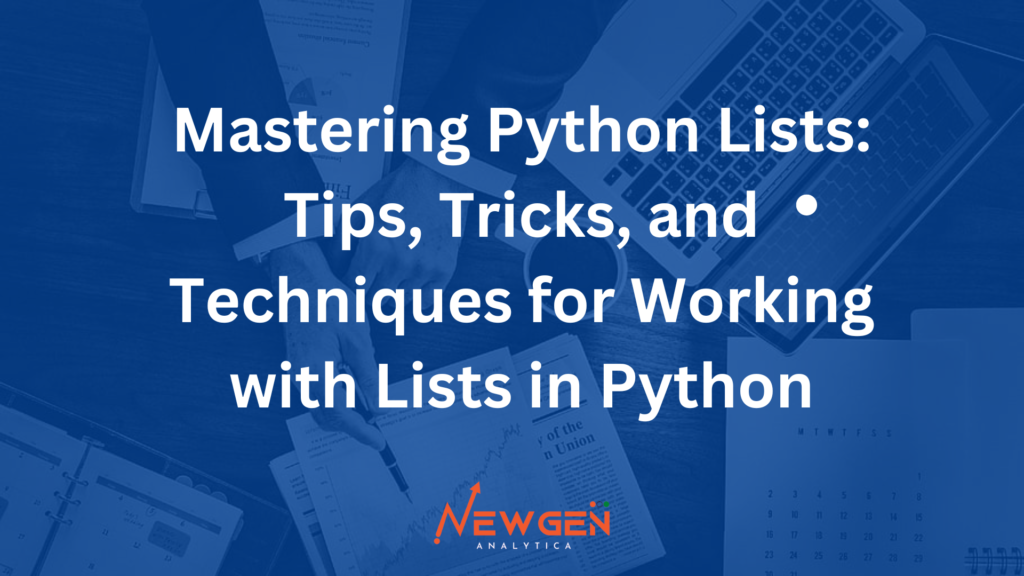
Mastering Python Lists: Tips, Tricks, and Techniques for Working with Lists in Python
Introduction to Python Lists
In Python, a list is a collection of items that are ordered and changeable. Lists are defined by square brackets [] and the items inside can be of any data type, such as integers, strings, or even other lists. Lists are commonly used to store and manipulate data in Python.
Here’s an example of a list:
fruits = ['apple', 'banana', 'orange']
Characteristics of Lists
In Python, lists have several characteristics that make them useful for different types of data manipulation and storage:
Ordered: Lists maintain the order of elements that are added to them, allowing you to access items by their index.
Changeable: Lists are mutable, meaning you can add, remove, and modify elements in a list.
Heterogeneous: Lists can contain elements of different data types, such as integers, strings, and other lists.
Indexing: Lists can be indexed, allowing you to access individual elements of a list by specifying their position in the list.
Slicing: Lists can be sliced, which means you can access a sub-list by specifying a start and end index.
Functions and methods: Lists have a variety of built-in functions and methods that can be used to perform operations on them, such as adding, removing, and sorting elements.
Iterable: Lists are iterable, so you can use them in for loops, list comprehension and other iterable context.
Pass by Reference: Lists are passed by reference and not by value, so any change made on list will reflect on the original copy of the list.
Indexing in Lists
In Python, lists are indexed, which means you can access individual elements of a list by specifying their position in the list. Indexing starts at 0, so the first element of a list is at index 0, the second element is at index 1, and so on.
You can access an element of a list using square brackets [ ] and the index of the element.
For example:
fruits = ['apple', 'banana', 'orange']
print(fruits[0]) # Output: 'apple'
print(fruits[1]) # Output: 'banana'
print(fruits[2]) # Output: 'orange'
You can also use negative indexing to access elements from the end of the list. The last element of a list is at index -1, the second-to-last element is at index -2, and so on.
fruits = ['apple', 'banana', 'orange']
print(fruits[-1]) # Output: 'orange'
print(fruits[-2]) # Output: 'banana'
print(fruits[-3]) # Output: 'apple'
You can also use indexing to modify an element of a list by assigning a new value to that index.
fruits = ['apple', 'banana', 'orange']
fruits[1] = 'mango'
print(fruits) # Output: ['apple', 'mango', 'orange']
It’s important to note that if you try to access an index that does not exist in the list, you will get an “IndexError” Exception.
Updating Lists
In Python, lists are mutable, which means you can update the elements of a list after it has been created. There are several ways to update elements in a list:
- Assignment: You can use indexing to change the value of an element in a list by assigning a new value to that index.
fruits = ['apple', 'banana', 'orange']
fruits[1] = 'mango'
print(fruits) # Output: ['apple', 'mango', 'orange']
- Append: The “append()” method allows you to add an element to the end of a list.
fruits = ['apple', 'banana', 'orange']
fruits.append('kiwi')
print(fruits) # Output: ['apple', 'banana', 'orange', 'kiwi']
- Insert: The “insert()” method allows you to add an element at a specific index in a list.
fruits = ['apple', 'banana', 'orange']
fruits.insert(1, 'mango')
print(fruits) # Output: ['apple', 'mango', 'banana', 'orange']
- Extend: The “extend()” method allows you to add multiple elements to a list at once.
fruits = ['apple', 'banana', 'orange']
new_fruits = ['mango', 'kiwi']
fruits.extend(new_fruits)
print(fruits) # Output: ['apple', 'banana', 'orange', 'mango', 'kiwi']
- Concatenation: You can concatenate two lists using the “+” operator.
fruits = ['apple', 'banana', 'orange']
new_fruits = ['mango', 'kiwi']
fruits += new_fruits
print(fruits) # Output: ['apple', 'banana', 'orange', 'mango', 'kiwi']
- Assignment with slices: You can also change multiple elements of a list by using slicing and assignment.
fruits = ['apple', 'banana', 'orange']
fruits[1:3] = ['mango','kiwi']
print(fruits) # Output: ['apple','mango','kiwi']
It’s important to note that when updating the list, you should be careful not to exceed the length of the list. When an index is not exist in the list, you will get an “IndexError” Exception.
Various List in-built functions
In Python, there are several built-in functions that can be used to perform operations on lists:
len(): The “len()” function returns the number of elements in a list.
fruits = ['apple', 'banana', 'orange']
print(len(fruits)) # Output: 3
max() and min(): The “max()” and “min()” functions return the maximum and minimum elements in a list, respectively. This only works for lists that contains elements that are comparable, otherwise it will raise an exception.
numbers = [1, 3, 2, 5, 4]
print(max(numbers)) # Output: 5
print(min(numbers)) # Output: 1
sorted(): The “sorted()” function returns a sorted copy of a list. It can take an optional argument “reverse=True” to sort the list in descending order.
fruits = ['orange', 'apple', 'banana']
print(sorted(fruits)) # Output: ['apple', 'banana', 'orange']
print(sorted(fruits, reverse=True)) # Output: ['orange', 'banana', 'apple']
sum(): The “sum()” function returns the sum of all elements in a list of numbers.
numbers = [1, 3, 2, 5, 4]
print(sum(numbers)) # Output: 15
count(): The “count()” method returns the number of occurrences of a specified element in a list.
fruits = ['apple', 'banana', 'orange', 'banana']
print(fruits.count('banana')) # Output: 2
index(): The “index()” method returns the index of the first occurrence of a specified element in a list.
fruits = ['apple', 'banana', 'orange']
print(fruits.index('banana')) # Output: 1
remove(): The “remove()” method removes the first occurrence of a specified element from a list.
fruits = ['apple', 'banana', 'orange']
fruits.remove('banana')
print(fruits) # Output: ['apple', 'orange']
pop(): The “pop()” method removes the element at the specified index, and returns the removed element. If no index is specified, it removes and returns the last element.
fruits = ['apple', 'banana', 'orange']
print(fruits.pop(1)) # Output: 'banana'
print(fruits) # Output: ['apple', 'orange']
reverse(): The “reverse()” method reverses the order of elements in a list.
fruits = ['apple', 'banana', 'orange']
fruits.reverse()
print(fruits) # Output: ['orange', 'banana', 'apple']
clear(): The “clear()” method removes all elements from a list.
fruits = ['apple', 'banana', 'orange']
fruits.clear()
print(
Summary
- Lists are a built-in data type in Python and are used to store an ordered collection of items.
- Lists are mutable, meaning they can be modified after they are created.
- Lists can hold items of any data type, including other lists.
- Lists can be indexed and sliced to access specific elements.
- Lists have various built-in methods such as append(), insert(), remove(), pop(), and sort().
- Lists can be concatenated and repeated using the + and * operators.
- Lists can be nested to create multidimensional lists.
- Lists can be used with loops and other control structures to perform various operations.
- Lists can be used with list comprehension to create a new list based on an existing one.
- Lists can be converted to other data types such as sets and tuples.
Top Common Questions related to lists
Q: How do I create a list in Python?
A: To create a list in Python, you can use square brackets [] and separate the items with commas. For example: my_list = [1, 2, 3, 4, 5]
Q: How do I access elements of a list in Python?
A: You can access elements of a list in Python by using indexing. The index of the first element is 0 and the index of the last element is -1. You can also use negative indexing to access elements from the end of the list. For example, my_list[0] will access the first element, my_list[-1] will access the last element.
Q: How do I add elements to a list in Python?
A: You can use the append() method to add elements to the end of a list, or the insert() method to add elements at a specific index. For example, my_list.append(6) will add the number 6 to the end of the list, my_list.insert(2, 7) will add the number 7 at the index 2.
Q: How do I remove elements from a list in Python?
A: You can use the remove() method to remove elements by value, or the pop() method to remove elements by index. For example, my_list.remove(6) will remove the first occurrence of the number 6 from the list, my_list.pop(2) will remove the element at index 2.
Q: How do I sort a list in Python?
A: You can use the sort() method to sort a list in ascending order. You can also pass the reverse=True argument to sort the list in descending order. For example, my_list.sort() will sort the list in ascending order, my_list.sort(reverse=True) will sort the list in descending order.
Q: How do I reverse a list in python?
A: You can use the reverse() method or slicing technique to reverse a list. For example, my_list.reverse() will reverse the list in place, my_list[::-1] will give you a new list which is reversed.
Q: How do I find the length of a list in Python?
A: You can use the len() function to find the length of a list. For example, len(my_list) will return the number of elements in the list.
Q: Can I use a for loop to iterate through a list in Python?
A: Yes, you can use a for loop to iterate through the elements of a list. For example:
for item in my_list:
print(item)
Q: Can I nest lists in python?
A: Yes, you can nest lists in python. It means you can have a list which contains other lists as its elements.
Conclusion
In conclusion, Python lists are a powerful and versatile data structure that can be used in a variety of ways. They offer many built-in methods for adding, removing, and manipulating elements, as well as slicing and indexing capabilities. Whether you are working with simple lists of numbers or more complex data structures, Python lists can help you organize and process your data effectively. With a solid understanding of how to use them, you can take your Python programming skills to the next level.